Computer Programming
A Wikibookian suggests that Competitive Programming be merged into this book. Discuss whether or not this merger should happen on the discussion page. |
Computer programming is the craft of writing useful, maintainable, and extensible source code which can be interpreted or compiled by a computing system to perform a meaningful task. Programming a computer can be performed in one of numerous languages, ranging from a higher-level language to writing directly in low-level machine code (that is, code that more directly controls the specifics of the computer's hardware) all the way down to writing microcode (which does directly control the electronics in the computer).
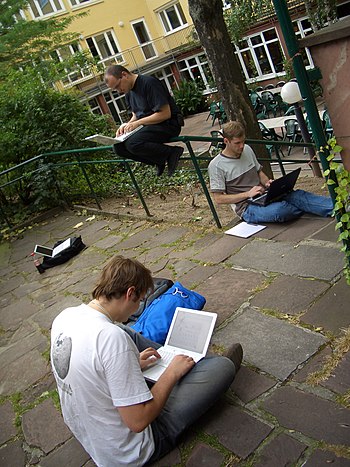
Using programming languages and markup languages (such as XHTML and XForms) require some of the same skills, but using markup languages is generally not considered "programming." Nevertheless, many markup languages allow inclusion of scripts, e.g. many HTML documents contain JavaScript. There are exceptions where markup languages do represent programming such as SuperX++ (http://xplusplus.sourceforge.net/) and o:XML (http://www.o-xml.org/)
Computer programming is one part of a much larger discipline known as software engineering, which includes several different aspects of making software including design, construction and quality control. The subject of this book is software construction, that is, programming. Computer programming is also a useful skill (though not always necessary) for people who are interested in computer science. Whereas software engineering is interested specifically in making software, computer science tends to be oriented towards more theoretical or mathematical problems.
Getting started
editMany people think they must choose a specific programming language in order to become a programmer, believing that they can only do that language. They ask themselves, "Should I be a C programmer or a Java programmer?" That's completely the wrong question. The right question is "How can I become a good programmer?" Unfortunately the employment market has contributed greatly to misconceptions about computer programming by companies advertising for employees with a specific (therefore limited) computer language skill-set and responses being handled by human resources(HR), without someone with a programming background.
There are a few points one can make about what a good programmer knows about specific computer languages. First - many languages are based on the same fundamental building blocks. Learning a language should be seen more as a way of acquiring those concepts than language or machine specific techniques. Second - good programmers are generally competent in more than one language because it is naturally interesting and useful to find different ways of solving problems. It is not necessary to master many different languages or even more than one—a programmer could excel in one language and have only a vague working idea how to program others. It is useful to know many different methods for solving computer problems, also known as algorithms. An algorithm is a list of well-defined instructions for completing a task, and knowing several languages means having the ability to list the computer instructions in many different ways. Since computer programming languages have so much in common, it is generally easy to learn a new programming language once you have mastered another.
So how do you get started? One reasonable technique would be to just pick a language and run with it. Unfortunately, we cannot suggest what the right computer language might be for all people for all purposes. Ask ten programmers what language you should learn and you will get ten different responses. Given the collaborative nature of this wikibook, you'll probably get as many responses as there are programming language books on the site.
Families of languages
editThere is a common misconception by people unfamiliar with computer programming that all programming languages are essentially the same. In one sense this is true because all digital electronic computers translate programming languages into strings of ones and zeros called binary, or Machine code.
While mainstream, personal computer languages tend to be derived from a specific tradition and are very similar (hence the popularity of this misconception), some languages fall into different paradigms which provide for a radically different programming experience. Programming in Java is quite different from programming in assembly language, which is quite different from programming in Haskell or Prolog or Forth, etc.
In the American Scientist article The Semicolon Wars[1], Brian Hayes classifies languages into four categories: imperative, object-oriented, functional, and declarative. Imperative and object-oriented languages tend to be used in the mainstream, whereas functional and declarative languages tend to be used in academic settings. Functional and declarative programming enthusiasts might argue that the paradigms are 20 years ahead of the mainstream and superior in many respects; however, mainstream language advocates would probably counter that such paradigms are hard to learn, or not very practical for their own unpopularity, among other things. We do not make any claims about who is right on this matter, but at the very least, we will suggest that building familiarity with the four major paradigms is an extremely valuable exercise.
- Functional programming
- Imperative programming
- (see also Procedural programming )
- Object oriented programming
When it comes to computers, all things are made, and function primarily by, programming. Although programming is an essential part of the functionality of any computer or application, not all programming languages are the same. In fact, they are very different from one another with different uses, functionality, and different levels of complexity. A programming language, in the most basic way, is a set of rules or guidelines that is used to write the computer programs. Even though you are writing the program, you may need a certain type of software or program for the language that you use. There are many different types of programming languages that can be used and each has a different set of rules. Programming has two basic categories. There are low-level and high-level languages, the difference between the two is that low-level languages often use 0s and 1s, and this works because it gives the computer the ability to quickly understand what needs to be done or executed. High level languages are easier to write because they are much closer to the English language and are much more flexible to write with, although there are also different levels of this readability as well and different categories of these languages that can be written. A few examples would be Visual Basic, C++, and Java.
Common concepts
editProgramming languages tend to have many general concepts in common. One can examine the recurring concepts and how they are expressed in various languages in the following table.
←
Table of language concepts and how they are expressed in various languages.
To see a comparison of syntax in various programming languages, see these "Hello World" examples. For a list including various computer languages arranged together by syntax terms and patterns, see Wikipedia's lists of computer syntax patterns.
Programming skills
editComputer programming is really just about solving problems. It turns out that a large number of the problems you encounter in the real world are really just special cases of a more general problem. Luckily for you, many of these problems have been studied by computer scientists for a very long time, sometimes leading to provably unbeatable solutions, or sometimes solutions which are "good enough" for every day needs. In short, learning a language gives you skills, but learning data structures and algorithms shows you how to use these skills wisely.
Data Structures and algorithms
editLanguage-related skills
edit- Agent Oriented Programming
- Ars based programming
- Aspect oriented programming
- Component based software development
- Concurrent programming
- Constraint-based programming
- Design by Contract
- Distributed programming
- Embedded programming
- Event driven programming
- Generic programming
- Post object programming
- Relational programming
- Symbolic programming
- System Programming
Programming for applications
edit- Application development
- Artificial Intelligence
- Databases
- Drivers
- Games
- Internet & Networking
- Multimedia
- Operating system creation
- Physics
Programming for platforms
editFunctionalities
editHistory of programming
editGeneral trends
edit- Procedural programming vs Declarative programming
- Structured programming (as opposed to unstructured programming)
Specific languages
editThe following languages deserve special mention, being significant languages in the development of structured programming languages and object-oriented programming. They are worth understanding for the concepts they introduced.
- ALGOL
- was the first structured programming language and as such is the basis for all modern imperative languages. It was built around the notion of a block, delimited by BEGIN and END containing declarations and statements. ALGOL is a recursive general-purpose language, including recursive calls at a time when this was thought too difficult in languages like FORTRAN and COBOL. ALGOL is a stack language, so as blocks are entered, the declared variables are pushed on the stack and when a block exits, the stack is reduced. A block without declarations is not actually a block, but a compound statement, causing no stack building overhead. ALGOL was first developed as ALGOL 58, based on the formal BNF (Backus-Naur Form) language design notation. ALGOL-60 was a revision and this formed the basis of many other languages such as ALGOL-68, ALGOL-W, Pascal, Modula-2, Oberon, C, C++, Java, Simula, Ada, and Eiffel. ALGOL-60 was the first language used for systems programming in the Burroughs B5000 stack machines operating system in the early 1960s (when all else was programmed in assembler and where ALGOL is still used in 2006 Current ALGOL manual ). Learning ALGOL will teach you the basis of block-structured languages and structured programming concepts.
- Simula
- was the first object-oriented language developed in 1967 using ALGOL-60 as a basis (much as C++ used C). It was originally conceived as a simulation language (hence the name) to model real world systems. As ALGOL had been a block-structured language with blocks entered on the stack as they were called and deleted from the stack when exited, Simula liberated programs from this restriction. Thus a block could be entered but when the code exited, the block persisted on the heap, rather than on the stack – thus the object was born. However, unlike records and structures (structs) of other languages, procedures were associated with this saved block so a programmer could define an entire algebra to manipulate entities. Thus variables declared as part of the outer block (class) persisted beyond the original invocation of the block code (which would now be considered a constructor or creation routine). Local variables and arguments to the procedures in the class would be pushed on the stack when called and popped when exited, but would manipulate the object’s persistent state. Class facilities could be abstracted by use of inheritance. Simula also included a sophisticated concurrency paradigm. Learning Simula will teach you object-oriented and simulation concepts along with concurrency concepts and the use of coroutines and cooperating processes.
- Smalltalk
- was developed by Adele Goldberg, Alan Kay (the real inventor of Windows) and others at Xerox PARC. It is an object-oriented language with a natural language syntax. It is a typeless language and thus very flexible and dynamic, resulting in quick turn around of code, but can result in exceptions at run time that would have been caught at compile time by a typed language. Its concepts were the basis of Objective-C. Learning Smalltalk will teach you object-oriented programming in a typeless language with a very different syntax.
- C++
- C++ (pronounced "see plus plus") is a general-purpose, object-oriented, statically typed, free-form, multi-paradigm programming language supporting procedural programming, data abstraction, and generic programming. During the 1990s, C++ became one of the most popular computer programming languages.
- Bjarne Stroustrup from Bell Labs was the designer and original implementer of C++ (originally named "C with Classes") during the 1980s as an enhancement to the C programming language. Enhancements started with the addition of classes, followed by, among many features, virtual functions, operator overloading, multiple inheritance, templates, and exception handling, these and other features are covered in detail in several Wikibooks on the subject.
- The C++ programming language standard was ratified in 1998 as ISO/IEC 14882:1998, the current version of which is the 2003 version, ISO/IEC 14882:2003.
- The 1998 C++ Standard consists of two parts: the Core Language and the Standard Library; the latter includes the Standard Template Library and C's Standard Library. Many C++ libraries exist which are not part of the Standard, such as Boost. Also, non-Standard libraries written in C can generally be used by C++ programs.
- Features introduced in C++ include declarations as statements, function-like casts, new/delete, bool, reference types, const, inline functions, default arguments, function overloading, namespaces, classes (including all class-related features such as inheritance, member functions, virtual functions, abstract classes, and constructors), operator overloading, templates, the :: operator, exception handling, run-time type identification, and more type checking in several cases. Comments starting with two slashes ("//") were originally part of BCPL, and was reintroduced in C++. Several features of C++ were later adopted by C, including const, inline, declarations in for loops, and C++-style comments (using the // symbol).
- Visual Basic
- Visual Basic is one of the worlds most commonly used programming languages. This is because of its simplicity and ease of use. The name BASIC stands for Beginners All-Purpose Symbolic Instruction Code and was developed at Dartmouth College in the USA for students. Visual Basic extends the functionality of the language so that creating windows, title-bars, input boxes, labels, message boxes and buttons is as simple as dragging the item you wish to code from a toolbar onto a form which is essentially a blank Window. One of the most basic functions of VB is the message box command. If I wanted to make a message box appear with a chosen message, all i would have to type would be: msgbox "hello world"
Additional Information
editEditors
editAn editor is simply a text program like Notepad. It is said that a programmer's best friend is the editor. A good editor is lightweight, has only essential tools and should support syntax highlighting for your language.
Examples of good editors for which we have teaching books are (alphabetically):
- Emacs
- An extensible, customizable, free/libre text editor — and more. At its core is an interpreter for Emacs Lisp, a dialect of the Lisp programming language with extensions to support text editing.
- Vim
- An abbreviation of Vi Improved, this editor is modelled on the classic Unix editor vi. However, Vim is a much more modern editor with an optional graphical interface and quite a few features vi doesn't have, making it the editor of choice for someone experienced with vi.
For more text editors, see Wikipedia's text editor category.
Tools
edit- gcc, a free compiler collection (Ada, C, C++, Fortran, Java, Objective-C, Objective-C++).
- make, the most popular build scripting language.
- Apache Ant, an XML-based build scripting language similar to Make.
- Some people use an integrated development environment (IDE) while programming.
- Eclipse, a software framework used as an IDE for Java, C++, PHP, Python, and a few other languages.
- Wikipedia:Comparison of integrated development environments lists an IDE for every popular programming language.
- Microsoft Visual Studio, an IDE (compiler included). Supporting C, C++, C++/CLI (via Visual C++), VB.NET (via Visual Basic .NET), C# (via Visual C#), and F# (as of Visual Studio 2010[9]). Support for other languages such as Python, Ruby, Node.js, and M among others is available via language services installed separately. It also supports XML/XSLT.
- Some people use a version control system. Such systems make it much easier to answer "Last week's version worked. This week's version doesn't. What changed?".
Popular libraries
editUnix native
Windows "native"
Cross platform
- Allegro
- wxWidgets
- OpenGL
- Qt - Popular object oriented C++ GUI framework. KDE is based on it. Bindings for various languages are available.
- XUL XML based GUI.
- Simple DirectMedia Layer (SDL)
Format Reference
editMisc
edit- Coding Style
- Debugging
- C Networking in UNIX
- An introduction to computer programming
- A beginners' programming forum
- Hello World Example Hello World Program Examples in Many Programming Language
Bibliography
edit- How to Design Programs
- Structure and Interpretation of Computer Programs (Videos)
- Wikisource: How to Think Like a Computer Scientist and its translation into Python, Think Python
- Software Engineering Body of Knowledge (SWEBOK)