Signetics 2650 & 2636 programming/2650 processor
The 2650 was first released by Signetics in 1975.[1] These video game consoles use the 2650A, a redesigned version that was smaller, cheaper to manufacture and had improved operating margins. The 2650A was released in 1977.[2] A later version, the 2650B, had a few design changes and additional features, but we aren't concerned with those here. The 2650 was fabricated in NMOS, was TTL compatible, and its many control signals made it simple to interface to. It was described by Adam Osborne as being a very minicomputer-like device having been closely based on the IBM 1130. It has a number of distinguishing features:
- 32k byte address range, organised as four 8k byte pages.
- Variable-length instructions of 1, 2 or 3 bytes.
- Single bit I/O path (flag and sense pins).
- Multiple addressing modes including indirect and indexed.
- Vectored interrupt.
- Eight-level return address stack.
- Seven 8-bit registers.
- Two program status bytes.
- Powerful instruction set.
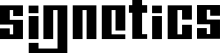
Registers
editThe 2650 has seven general purpose 8-bit registers. R0 is always available, whereas there are two banks of R1, R2 and R3 which are selected by the RS bit in the Program Status Word.
Program Status Word
editThe Program Status Word (PSW) is a special purpose register that contains status and control bits. It is divided into two bytes, the Program Status Upper (PSU) and Program Status Lower (PSL). The PSW bits may be tested, loaded, stored, preset or cleared using the instructions tpsu, tpsl, lpsu, lpsl, spsu, spsl, ppsu, ppsl, cpsu or cpsl.
|
| ||||||||||||||||||||||||||||||||||
|
Reset
editWhen a Reset pulse is applied to the 2650A, program execution is forced to begin at memory address 0. The only other thing we know for sure about the state of the processor after reset is that the Interrupt Inhibit bit in the Program Status Word is cleared. As we very much don’t want spontaneous interrupts to happen, one of the first things our program should do is set it to disable them until we ready for them. The “Signetics 2650 Initialization Application Memo” also suggests that we may set the Stack Pointer to zero, and that we should set the Register Select bit to a known state.[5]
The With Carry bit and the Compare bit may be set to whatever condition you will need them in first. In a simple program which has them in the same state throughout, this is a straightforward decision. In more complex programs it is generally best to set them to the most used condition initially, and then switch them back and forth whenever the opposite condition is needed. If the With Carry bit is on, then the Carry bit should be initialised appropriately before the first arithmetic instruction. Otherwise there is no need to initialise Carry or any of the other bits (Condition Code, Interdigit Carry, or Overflow). [5]
Interrupts
editCertain events occurring in the PVI cause it to signal the 2650 that it needs attention by setting the interrupt line low. If the Interrupt Inhibit bit in the Program Status Word is not set, (in other words interrupts are enabled), the processor will finish the current instruction and then set the Interrupt Inhibit bit. The PVI then outputs its interrupt vector, 3, to the data bus and the processor executes a ZBSR (branch to subroutine on page zero) to address $0003.
When the interrupt service subroutine has finished, it should be exited with either a RETC or RETE instruction. The latter will re-enable interrupts.
Instruction set
editAll mnemonics in the 2650A instruction set are 3 or 4 characters long. The first two or three characters specify the operation, and if present, a final character indicates an addressing mode to use (see the next section, Addressing modes for more detail). Assembly code for the 2650 generally looks something like this:
loop: lodi,r0 $20 ; load r0 from the byte immediately following, $20 stra,r0 $1F40 ; store r0 in the absolute address $1F40 eorz r0 ; exclusive-or r0 with itself bctr,un loop ; branch relative, unconditionally, to address specified by 'loop'
There are 34 basic instructions:
Transfer | LOD | Z,I,R,A | Load register |
STR | Z,R,A | Store register | |
Arithmetic | ADD | Z,I,R,A | Add to register |
SUB | Z,I,R,A | Subtract from register | |
DAR | Decimal adjust register | ||
Logical | AND | Z,I,R,A | Bitwise AND to register |
IOR | Z,I,R,A | Bitwise OR to register | |
EOR | Z,I,R,A | Bitwise EXCLUSIVE-OR to register | |
Test | COM | Z,I,R,A | Compare value to register |
TM | I | Test masked bits of register | |
Rotate | RRR | Rotate register right by one bit | |
RRL | Rotate register left by one bit | ||
Branch | BCT | R,A | Branch on condition true |
BCF | R,A | Branch on condition false | |
BRN | R,A | Branch if register not-zero | |
BIR | R,A | Increment the register and branch if it is not zero | |
BDR | R,A | Decrement the register and branch if it is not zero | |
ZBR | R | Branch, relative to address $0000 | |
BX | A | Branch, indexed | |
Call / Return | BST | R,A | Branch to subroutine on condition true |
BSF | R,A | Branch to subroutine on condition false | |
BSN | R,A | Branch to subroutine if register not-zero | |
ZBS | R | Branch to subroutine, relative to address $0000 | |
BSX | A | Branch to subroutine, indexed | |
RET | C,E | Conditionally return from subroutine. RETE also enables interrupts | |
Input / Output | WRT | C,D,E | Input/output hardware is not implemented on these consoles |
RED | C,D,E | ||
Miscellaneous | HALT | Enter WAIT state until processor is reset or interrupted | |
NOP | Do nothing except fetch and execute this instruction | ||
Program status word | LPS | U,L | Load the PSW |
SPS | U,L | Store the PSW | |
CPS | U,L | Clear specified bits in the the PSW | |
PPS | U,L | Preset specified bits in the the PSW | |
TPS | U,L | Test specified bits in the the PSW |
Addressing modes
editThe Signetics 2650 has four main addressing modes: Register, Immediate, Relative and Absolute. In addition Indirect and Indexing may be combined with some of these.
Register addressing
editAll register-to-register instructions are 1 byte in length and use R0 as the first operand, while the other can be any of R0, R1, R2, R3.
lodz r3 ; load r0 from r3 addz r2 ; r0 = r0 + r2 lodz r0 ; not very useful! eorz r0 ; exclusive-or r0 with itself ; very useful! A one byte instruction for r0 = 0
Immediate addressing
editIn immediate addressing the first operand, which can be any register, is specified next to the instruction; followed by the value of the other operand, which implicitly must be a constant. All immediate addressing instructions are two bytes in length.
lodi,r2 $20 ; r2 = 32 subi,r0 8 ; r0 = r0 - 8 iori,r1 %00100000 ; set bit5 of r1 comi,r3 15 ; compare r0 to 15
Absolute addressing
editIn absolute addressing the first operand, which can be any register(but see exception in Indexed addressing below), is specified next to the instruction. The second argument designates the absolute address of the memory location where the other operand is located.
Relative addressing
editIn relative addressing the first operand, which can be any register, is specified next to the instruction. The second argument designates a memory location where the other operand is located. This second argument is a relative displacement from the current address and can be any value from -64 to +63. In practice we don't specify this value as a number. It generally takes the form of a label and the assembler does the calculation for us. If the label is too far away the assembler will generate an error message. Relative addressing is also used in branch instructions to jump to a memory location close by.
lodr,r2 number ; r2 = 42 bctr,un next ; branch to next number: db 42 ; define a data byte = 42 next: addi,r2 53
Indexed addressing
editWhen indexed addressing is used, the effective address is calculated by adding the contents of the specified index register to the address field. The addition uses the value in the index register as an 8-bit positive number.
lodi,r2 5 loda,r0 $1F00,r2 ; r0 = contents of address $1F05
Note that the first argument is always r0. Some assemblers may allow this to be omitted from the code, but for the sake of readability it should be included if possible.
Two other options allow for the index register to be auto-decremented or auto-incremented before it is added to the base address:
lodi,r2 5 loda,r0 $1F00,r2+ ; r0 = contents of address $1F06 lodi,r3 $A6 loda,r0 $1000,r3- ; r0 = contents of address $10A5
Indexing may only be used with absolute addressing. It may not be used with branch instructions, but two special instructions exist for this purpose. They are BXA (branch indexed, absolute) and BSXA (branch to subroutine indexed, absolute). Auto-increment and auto-decrement cannot be used with them, and register R3 must be specified as the index register.
Indirect addressing
editIndirect addressing means that the argument address of an instruction is not specified by the instruction itself, but rather the argument address will be found in the two bytes pointed to by the instruction. The indirect addressing mode is indicated by an asterisk.
pointer dw $0F00 ; in most cases this would be an address in RAM, i.e. a variable ..... loda,r1 *pointer ; r1 = contents of $0F00
Indirection may be used with relative addressing as well, but since code in these consoles is operating out of ROM, and RAM addresses are generally too far away for a relative address, there is no apparent use. One exception does occur when space for code is at a premium:
:bar equ $1F0E 0000 066A : lodi,r2 $6A 0002 CE1F0E : stra,r2 bar 0005 0D1F0E :foo loda,r1 bar 0008 0BFC : lodr,r3 *foo+1 ; load r3 indirectly from $0006, i.e. $1F0E
The last two instructions both load a register from bar, but the last instruction only uses two bytes rather than three.
Indexed Indirect addressing
editWhen indexing and indirect are used together, the value of the index register is added to the indirect address, not to the value in the address field of the instruction.
Further reading
editFor more detailed information consult the Signetics 2650 User Manual, available as a full pdf or abridged for programmers as html.
References
edit- ↑ Microcomputer Digest (PDF). Cupertino, CA: Microcomputer Associates Inc. 2 (1): 1–3. July 1975 http://bitsavers.trailing-edge.com/magazines/Microcomputer_Digest/Microcomputer_Digest_v02n01_Jul75.pdf. Archived (PDF) from the original on 1 February 2014. Retrieved 4 December 2021.
{{cite journal}}
: Cite has empty unknown parameter:|1=
(help); Invalid|url-access=4 December 2021
(help); Missing or empty|title=
(help) - ↑ "Signetics 2650: An IBM on a Chip". The CPUshack museum. October 16th, 2016. Retrieved 5 December 2021.
{{cite web}}
: Check date values in:|date=
(help) - ↑ Osborne, Adam (1978). An introduction to microcomputers (PDF). Berkeley, Calif.: Osborne & Associates. p. 736. ISBN 0-931988-15-2. Retrieved 4 November 2021.
- ↑ 2650 Series Microprocessor datasheet (PDF). Philips. Retrieved 4 November 2021.
- ↑ a b Signetics Applications Memo MP51 - 2650 initialization (PDF). Philips. Retrieved 4 November 2021.